Databases are an essential part of computer applications. There are many kids of databases and they can be quite challenging to understand and learn. TinyDB provides a simple and easy way to learn the basics of databases with Python. This knowledge is directly applicable even with other databases such as SQlite and MongoDB.
What is a Database?
A database is a way to store data in an organized way such that it persists even when our program is not running and becomes available when the application is used.
It also allows us to easily and efficiently add, retrieve and search for information even when there are very large quantities of data. Moreover, it gives us the ability to secure and access data safely with encryption and authentication.
Why Do We Need a Database?
We need a database when we have persistent data that is required for an application. Efficient storage and searching are one of the main requirements from a database. The ability to add security and have multiple levels of access are some of the more important reasons databases are employed in applications.
What is TinyDB?
TinyDB is a very small implementation of a document-oriented database (similar to MongoDB) which can be employed for small applications. In our case, we use it as a stepping stone to learning about databases and utilize it in small applications.
It is written purely in Python (a useful resource for some of the built-in features of Python can be found here: https://www.youngwonks.com/resources/python-cheatsheet) and has a very simple Application Programming Interface (API). It is also fun to work with as it simpler than the programming language Structured Query Language (SQL). TinyDB is also well documented on Read the Docs (an open-sourced free software documentation hosting platform) and doesn’t need a lot of configurations. It is easy to hit the ground running. Given that it is open source, the source code can be viewed on Github.
When not to use TinyDB?
TinyDB is not suitable for large applications that mandate high performance. It also doesn’t support parallel connections to the database. For such needs, we should turn to MongoDB, SQlite or MySQL, among many others.
Basic Database Operations – CRUD
Each database provides four basic operations that form the basis for other operations performed on the database. These operations are often described by the abbreviation CRUD – CREATE READ UPDATE DELETE.
- CREATE – The CREATE operation lets the user create new tables in the database and insert new records into the table.
- READ – The READ operation allows the user to retrieve records from the database.
- UPDATE – The UPDATE operation allows the user to modify the information stored in the database.
- DELETE – The DELETE operation allows the user to remove the records from the database.
In this tutorial, we will take a look at the basic usage of each of these operations.
Installation and Import
Tiny DB is available on Python Package Index (PyPi), the official third-party software repository for Python and is very easy to install as shown below:
- Windows:
- pip install tinydb
- Linux & Mac:
- pip3 install tinydb
In order to check your installation, create a new file and add the line shown below,
Syntax:

Create
The create operations involves, creating a database followed by creating an entry in the database. Here we initialize the TinyDB object with a parameter specifying the name of the file in which we wish to store our information. The data is stored in the JSON (JavaScript Object Notation) file format; this is a lightweight data-interchange format.
We use the insert method (db.insert) to add an entry to the Tinydb database. The general format to be added is similar to a dictionary, where we specify key value pairs. The key-value pairs serve as the field names and their corresponding values within a TinyDB document.
Syntax:
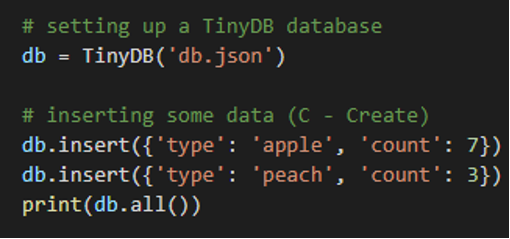
Read
Reading data from the data involves being able to read the entire database(db.all), reading or searching for a specific entry (db.search) and also being able iterate over multiple entries one by one.
Syntax:
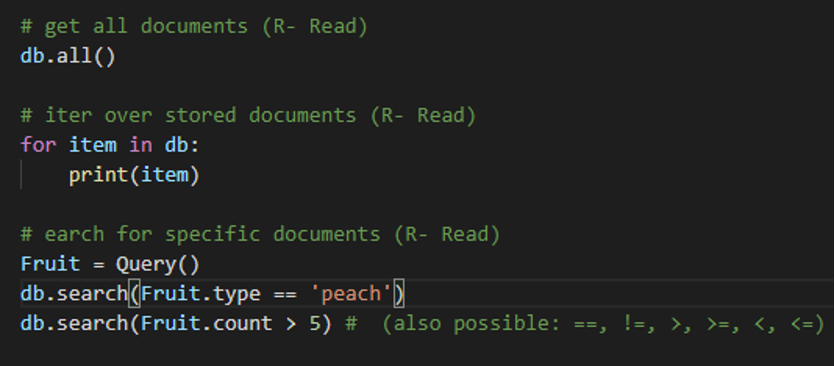
Update
The update operation (db.update) involves being able to update all entries in the database and also being able to search for and update entries.
Syntax:
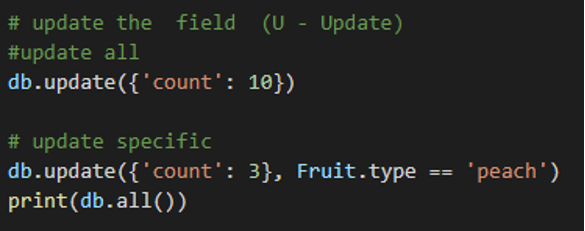
Delete
The delete operation (db.remove) allows us to search and delete specific entries or completely erase the contents of the database.
Syntax:
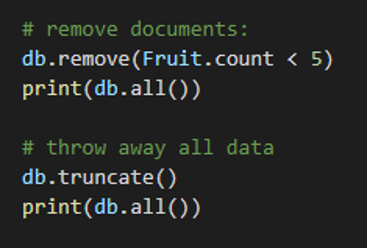
Further
In order to sharpen our skills and work our way towards advanced usage and other sophisticated databases, it may be a good idea to work on the following projects:
- Try a text-based Python login system which stores usernames and passwords in TinyDB.
- Use TinyDB to store a list of words in your Hangman game.
- Use TinyDB for the leaderboard in your Pygame.